Hello all, My program is a guessing game that allows the user to enter the low and high numbers and how many guesses he/she gets. Then the program generates a random number between the low and high. Got everything working fine, just some small things that I need help with and one big thing that I am confused on.
SMALL THINGS:
1) When they incorrectly guess the number it tells you then says how many guesses you have left on your last guess it prints "Incorrect guess. You have 0 guess left". I don't want it to print that. Just want you to enter you last guess and if its wrong it just say " You lost!!!".
2) I need to have the while to evaluate a CHAR of either Y/N but I couldn't get it to work. I just threw in a int just to see if it was even capable of working, it was. I had it as String line = nextLine(); but when I had this in it didn't even allow me to type anything.
3) If you say yes to continue and you reenter the same low and high numbers the random number is always the same. For example I ran it 4 times with the low = 1 and high = 3 and the random number was always 3. Is there a way to make it randomize a new number every time they say yes to playing again?
BIG THING:
"GameInfo class. Your program should contain a class called GameInfo that will be used to store the following data during program execution: low and high range values, number of guesses allowed, number of guesses taken, did the player win or lose. Make sure you write get/set methods and at least one constructor."
I know how to make the new class and stuff. Just confused on how I would get the values in the guess class over to the GameInfo class so I can print to a file.
The files format is like this:
Won Hgh Low Alw Tkn
I need to put in the info for every time they play game until the program ends.
What it should look like:
Thanks to all that help =D
SMALL THINGS:
1) When they incorrectly guess the number it tells you then says how many guesses you have left on your last guess it prints "Incorrect guess. You have 0 guess left". I don't want it to print that. Just want you to enter you last guess and if its wrong it just say " You lost!!!".
2) I need to have the while to evaluate a CHAR of either Y/N but I couldn't get it to work. I just threw in a int just to see if it was even capable of working, it was. I had it as String line = nextLine(); but when I had this in it didn't even allow me to type anything.
3) If you say yes to continue and you reenter the same low and high numbers the random number is always the same. For example I ran it 4 times with the low = 1 and high = 3 and the random number was always 3. Is there a way to make it randomize a new number every time they say yes to playing again?
BIG THING:
"GameInfo class. Your program should contain a class called GameInfo that will be used to store the following data during program execution: low and high range values, number of guesses allowed, number of guesses taken, did the player win or lose. Make sure you write get/set methods and at least one constructor."
I know how to make the new class and stuff. Just confused on how I would get the values in the guess class over to the GameInfo class so I can print to a file.
The files format is like this:
Won Hgh Low Alw Tkn
I need to put in the info for every time they play game until the program ends.
Code:
import java.util.Scanner;
import java.io.PrintStream;
import java.util.Random;
public class Guess {
public static void main(String[] args) {
int high;
int low;
int guess;
int guesses;
int rNum;
int g;
Scanner input = new Scanner(System.in);
System.out.println("Number Guessing Program");
System.out.println("------------------------\n");
do
{
System.out.printf("\nEnter the low value: ");
low = input.nextInt();
System.out.printf("Enter the high value: ");
high = input.nextInt();
System.out.printf("Enter the number of guesses allowed: ");
guesses = input.nextInt();
Random rand = new Random();
rNum = rand.nextInt(high-low) + (low+1);
for (int c = 0; guesses > c; guesses--)
{
System.out.printf("Enter a guess (%d-%d): ",low,high);
guess = input.nextInt();
if (guess != rNum)
{
System.out.printf("Incorrect guess. You have %d guess left\n",(guesses-1));
}
else
{
System.out.printf("You won!!!\n\n");
break;
}
if (guesses == 1)
System.out.printf("You lost!!!");
}
System.out.printf("\nDo you want to keep playing (Y/N): ");
g = input.nextInt();
} while ((g == 1) || (g == 1));
System.out.println("\nGame over. Thank you\n\n");
}
}
What it should look like:
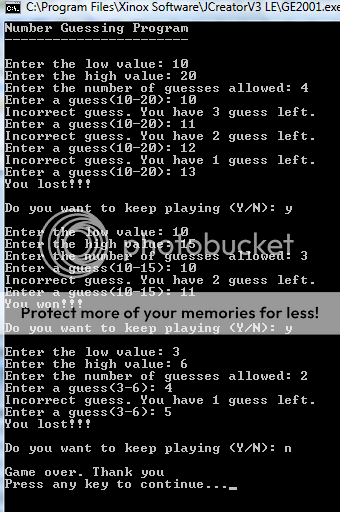
Thanks to all that help =D
